Data is very important for businesses because it helps make better decisions. Extracting data from Sitecore is essential since it contains valuable information. As a developer you will often get requests from business to export data from Sitecore. There are many ways to do it and in this example I am going to explain how you can do it with a standalone aspx page without requiring any deployment.
The thing I love most about this technique is that you can write simple .NET code and call your internal libraries or create business logic on the fly to get reports and export whatever you need from Sitecore. By leveraging ASP.NET’s powerful features, developers maintain control over data export processes, optimizing performance and ensuring efficient data retrieval even for large datasets.
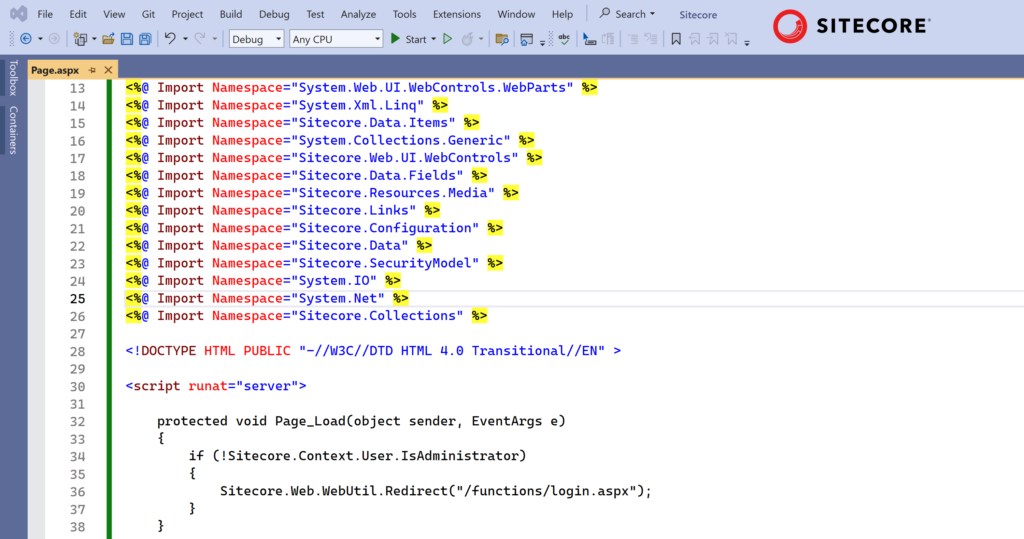
ASP.NET Example to Export Sitecore Item Data
Please note this example, simply outputs data to the page as HTML table, you can always copy paste into Word/Excel or other tools. You will need to have Sitecore admin access to run this.
You can also use code-behind i.e. but that require deployment where-as this method gives you more flexibility.
<%@ Page Language="c#" %>
<%@ Import Namespace="System" %>
<%@ Import Namespace="System.Collections" %>
<%@ Import Namespace="System.Configuration" %>
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.Linq" %>
<%@ Import Namespace="System.Web" %>
<%@ Import Namespace="System.Web.Security" %>
<%@ Import Namespace="System.Web.UI" %>
<%@ Import Namespace="System.Web.UI.HtmlControls" %>
<%@ Import Namespace="System.Web.UI.WebControls" %>
<%@ Import Namespace="System.Web.UI.WebControls.WebParts" %>
<%@ Import Namespace="System.Xml.Linq" %>
<%@ Import Namespace="Sitecore.Data.Items" %>
<%@ Import Namespace="System.Collections.Generic" %>
<%@ Import Namespace="Sitecore.Web.UI.WebControls" %>
<%@ Import Namespace="Sitecore.Data.Fields" %>
<%@ Import Namespace="Sitecore.Resources.Media" %>
<%@ Import Namespace="Sitecore.Links" %>
<%@ Import Namespace="Sitecore.Configuration" %>
<%@ Import Namespace="Sitecore.Data" %>
<%@ Import Namespace="Sitecore.SecurityModel" %>
<%@ Import Namespace="System.IO" %>
<%@ Import Namespace="System.Net" %>
<%@ Import Namespace="Sitecore.Collections" %>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN" >
<script runat="server">
protected void Page_Load(object sender, EventArgs e)
{
if (!Sitecore.Context.User.IsAdministrator)
{
Sitecore.Web.WebUtil.Redirect("/functions/login.aspx");
}
}
protected void GoButton_Click(object sender, EventArgs e)
{
try
{
var path = "/sitecore/content/home/Products";
int rowNumber = 1;
Database master = Sitecore.Configuration.Factory.GetDatabase("master");
Item rootItem = master.GetItem(path, Sitecore.Context.Language);
if (rootItem == null) throw new Exception(path + " does not exist");
var allItems = rootItem.Axes.GetDescendants().OrderBy(x => x.Paths.FullPath);
if (allItems == null || allItems.Count() <= 0) throw new Exception("No Items under this folder");
Response.Write("<table border='1'>");
foreach (var item in allItems.Where(x => x.TemplateName.Equals("ParentProduct")))
{
try
{
Response.Write("<tr>");
Response.Write("<td>" + rowNumber + "</td>");
Response.Write("<td>" + item.ID.ToString() + "</td>");
Response.Write("<td>" + item.DisplayName + "</td>");
Response.Write("<td>" + item["shortDescription"] + "</td>");
Response.Write("<td>" + item["additionalDescription"] + "</td>");
Response.Write("<td>" + item.Paths.FullPath + "</td>");
Response.Write("</tr>");
}
catch (Exception ex)
{
Response.Write("Error : " + ex.Message + "");
}
rowNumber++;
}
Response.Write("</table>");
}
catch (Exception ex)
{
Response.Write(ex.ToString() + ex.StackTrace.ToString());
}
}
</script>
<html>
<head>
<title>Item Report</title>
</head>
<body>
<form id="Form2" method="post" style="font-weight: bold" runat="server">
<br />
<asp:CheckBox ID="ShowEmptyCheckBox" Checked="true" Text="Show only empty results" runat="server" />
<br />
<asp:Button ID="GoButton" runat="server" Text="Show Report" OnClientClick="return confirm('Are you sure?');"
OnClick="GoButton_Click" />
<br />
<br />
<br />
</form>
</body>
</html>
https://github.com/zaheer-tariq/Sitecore-Blog-Gists/blob/main/Pages/Reports/ItemReport.aspx
How to upload and test this ASPX page
You can use Visual Studio to create and test this page in your local solution. You can copy the page to Sitecore Web Application in Internet Information Services (IIS) to /sitecore/admin/ or any other directory and access it through your CM URL as https://your-cm-hostname/sitecore/admin/ItemReport.aspx
In production or other environments where you may not have access to Sitecore directly, you can use Sitecore File Explorer tool https://your-cm-hostname/sitecore/shell/default.aspx?xmlcontrol=FileExplorer&sc_lang=en
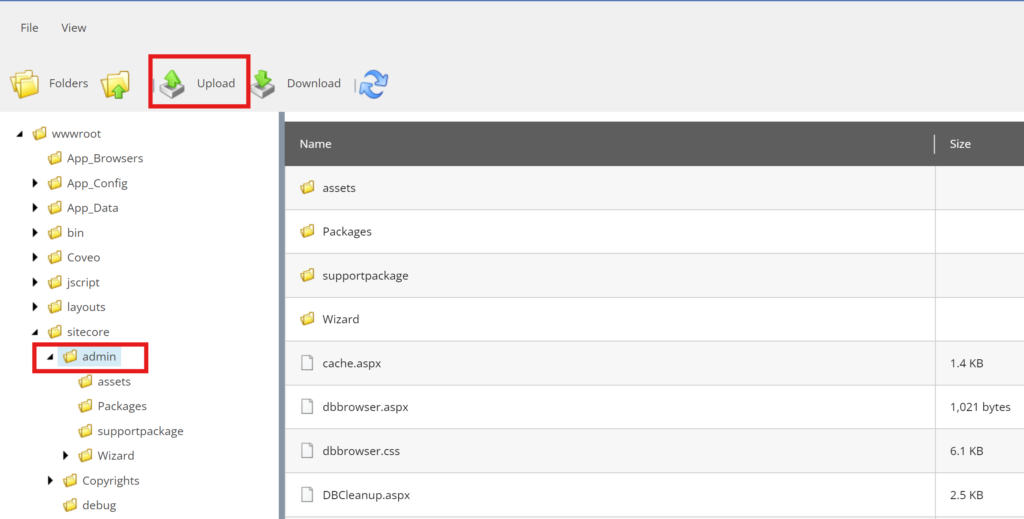
Keywords:
Sitecore data export, ASP.NET ASPX pages, Sitecore API integration, data retrieval, data formatting, content management, digital experience, ASP.NET development, custom data export, data-driven decision-making.
Hashtags:
#Sitecore #ASPdotNET #DataExport #ContentManagement #DigitalExperience #APIIntegration #DataRetrieval #ASPXPages