If you’re looking for an API endpoint implementation that can take a dynamic path from a query string to revalidate page cache and force page regeneration, you’ve come to the right place.
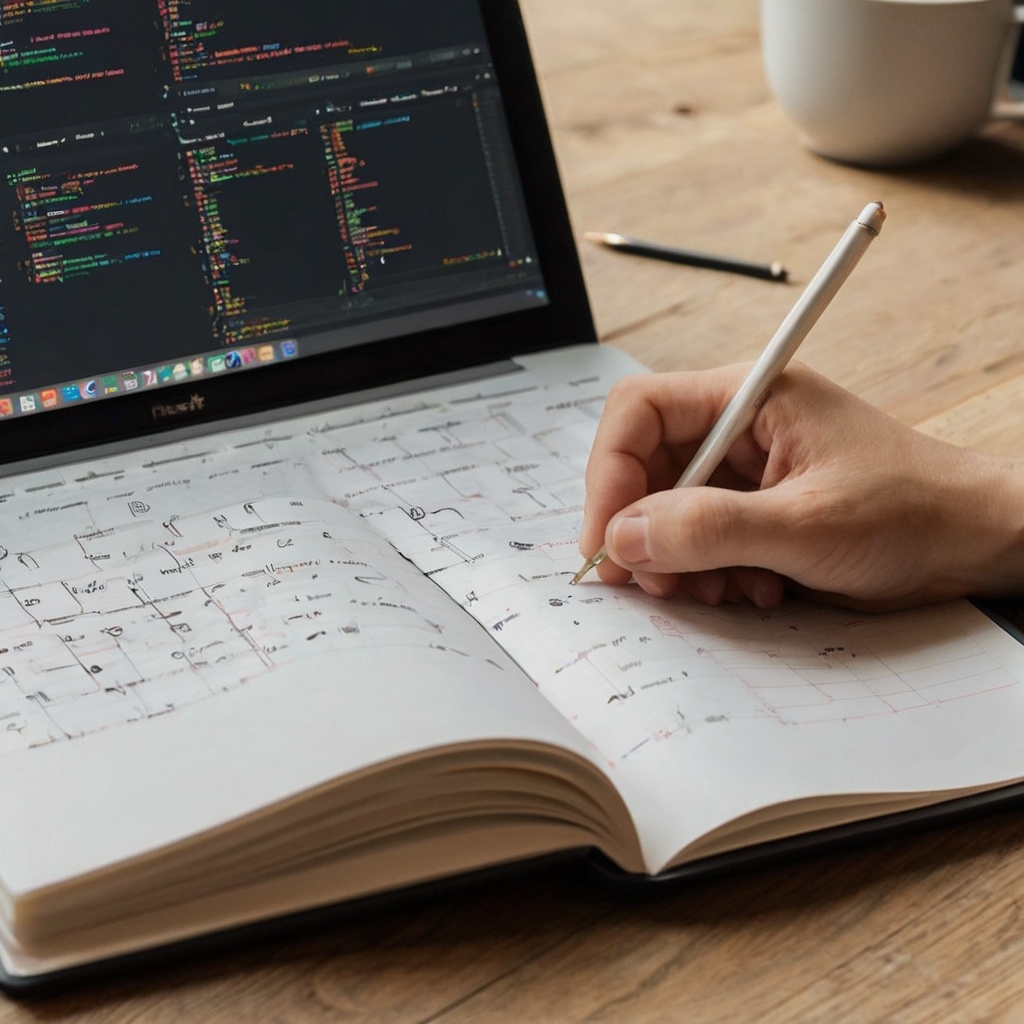
The biggest challenge with using statically generated sites is the difficulty of pushing content updates quickly in urgent situations. You can’t set the revalidation value too low because it will increase the number of API calls to your GraphQL endpoint and could make the entire architecture impractical if pages are regenerated too often.
In modern web development, ensuring up-to-date content and optimal performance is crucial. When building a Sitecore website using Next.js with Incremental Static Site Generation (ISSG), and deploying it on Vercel, on-demand cache revalidation becomes essential. This article will show you how to implement on-demand cache revalidation in a Next.js app using only the pages
folder or only app
folder, with TypeScript.
Creating a Get API Endpoint for On-Demand Page Cache Revalidation
To handle revalidation requests, at run time the example below takes a secret and page path from query string and triggers page cache revalidation at runtime. Advantage of having this as a Get call is that you can pass it to non-technical content editors and they can themselves clear the cache if needed. The output will also render the page link so you can click and view the update page.
Pages folder API Endpoint for On-Demand Page Cache Revalidation
import { NextApiRequest, NextApiResponse } from 'next';
export const handler = async (req: NextApiRequest, res: NextApiResponse): Promise<void> => {
const publicUrlHost = process.env.VERCEL_ENV_URL
? `https://${process.env.VERCEL_ENV_URL}`
: process.env.PUBLIC_URL;
const { secret, path } = req.query;
if (secret == null) {
return res.status(400).send('secret is required');
}
if (path == null) {
return res.status(400).send('path is required');
}
// Check for secret token
if (secret !== process.env.JSS_REVALIDATE_SECRET) {
return res.status(400).send('Invalid secret');
}
try {
// this should be the actual path not a rewritten path
const pagePath = path.toString();
await res.revalidate(pagePath);
const pageUrl = publicUrlHost + pagePath;
return res.status(200).send(`
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Cache Clear</title>
</head>
<body>
<h4>Cache cleared for: <a href="${pageUrl}">${pageUrl}</a></h4>
</body>
</html>
`);
} catch (err) {
// If there was an error, Next.js will continue to show the last successfully generated page
return res.status(500).send('Error revalidating:' + err);
}
};
export default handler;
https://github.com/zaheer-tariq/Sitecore-Blog-Gists/blob/main/NextJs/pages/api/devops/revalidate.ts
App folder API Endpoint for On-Demand Page Cache Revalidation
import { revalidatePath } from 'next/cache';
import { NextRequest, NextResponse } from 'next/server';
export async function GET(request: NextRequest): Promise<NextResponse> {
const secret = request.nextUrl.searchParams.get('secret');
const path = request.nextUrl.searchParams.get('path');
if (secret == null) {
return NextResponse.json({ message: 'secret is required' });
}
if (path == null) {
return NextResponse.json({ message: 'path is required' });
}
// Check for secret token
if (secret !== process.env.JSS_REVALIDATE_SECRET) {
return NextResponse.json({ message: 'Invalid credentials' });
}
if (path) {
revalidatePath(path);
return NextResponse.json({ message: 'Cache cleared for: ' + path });
}
return NextResponse.json({ message: 'Invalid status' });
}
https://github.com/zaheer-tariq/Sitecore-Blog-Gists/blob/main/NextJs/app/api/revalidate/route.ts
Keywords: API endpoint implementation, dynamic path query string, revalidate page cache, force page regeneration, statically generated sites, content updates, revalidation value, GraphQL endpoint, site architecture, modern web development, up-to-date content, optimal performance, Sitecore website, Next.js, Incremental Static Site Generation (ISSG), Vercel deployment, on-demand cache revalidation, TypeScript, Get API endpoint, page cache revalidation, runtime revalidation, non-technical content editors, clear cache, view updated page